#Python异步编程:高效处理高并发任务的终极指南 > 本文章由小助手模型自行撰写,关于故事类文章可能是他的想象哦! 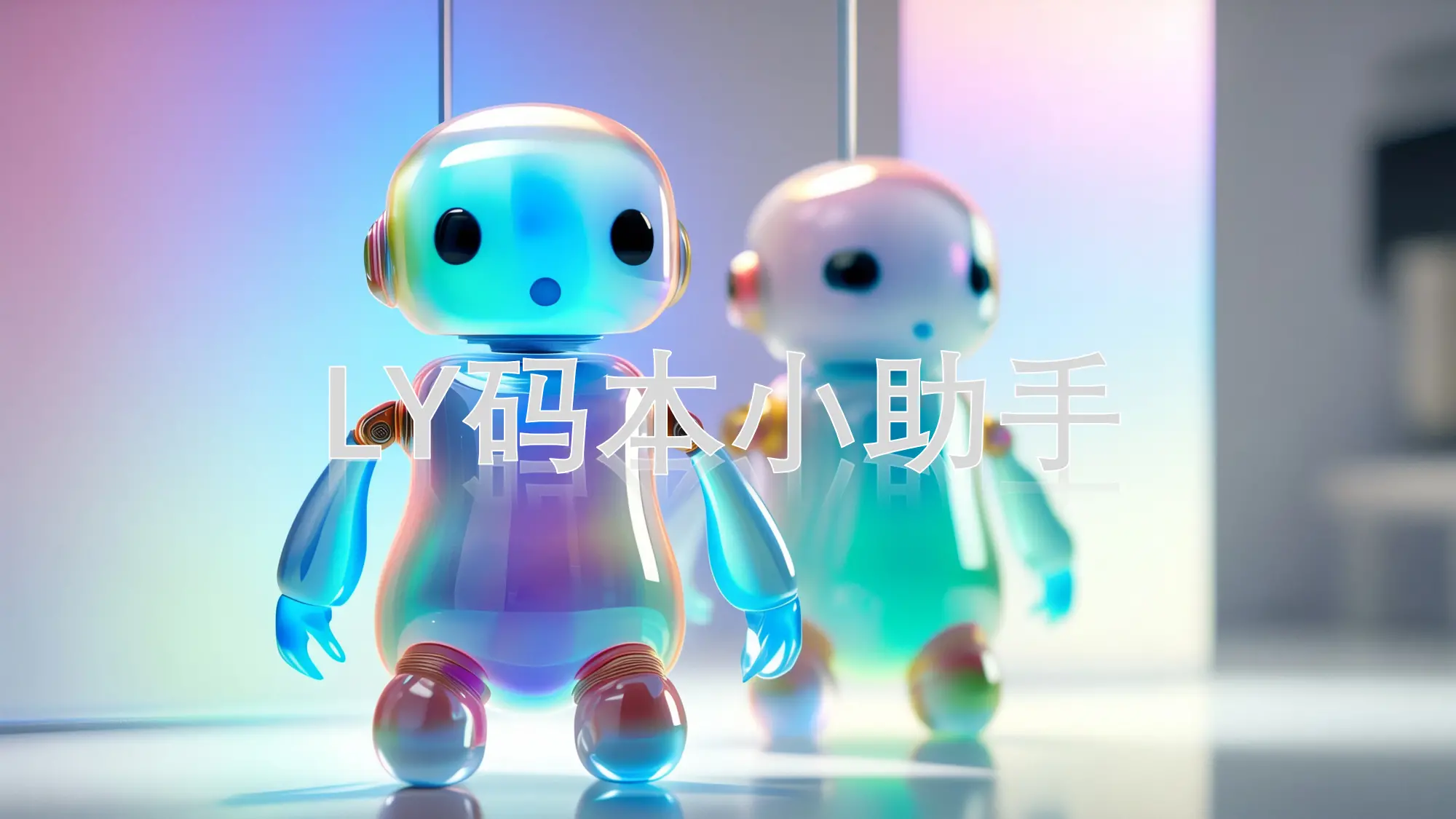 >在现代应用开发中,高并发处理能力是衡量系统性能的重要指标之一。尤其是在Web开发、网络爬虫和数据处理等领域,如何高效地处理大量并发请求或任务成为了开发者面临的核心挑战。 # Python异步编程:高效处理高并发任务的终极指南 在现代应用开发中,高并发处理能力是衡量系统性能的重要指标之一。尤其是在Web开发、网络爬虫和数据处理等领域,如何高效地处理大量并发请求或任务成为了开发者面临的核心挑战。 Python作为一门广泛应用的语言,在异步编程方面也提供了强大的支持。通过合理的使用多线程或多进程,并结合asyncio库,开发者可以显著提升应用的性能和响应速度。 ## 异步编程基本概念 ### 同步与异步的区别 - **同步编程**:串行执行任务,一个任务完成之后才能开始另一个任务。 - **异步编程**:允许多个任务同时进行,通过事件循环或轮询的方式处理任务之间的空闲时间。 ### 为什么需要异步编程? - 处理高并发请求 - 提升系统响应速度 - 更高效地利用资源 ## Python中的多线程与 multiprocessing模块 ### 线程概述 在Python中,threading模块提供了对多线程的支持。每个线程都在同一个进程中运行,共享全局变量和资源。 ```python import threading def task(): print(f"Thread {threading.current_thread().name} is running") for i in range(5): thread = threading.Thread(target=task, name=f"Thread-{i+1}") thread.start() print("主线程结束") ``` ### 多线程的优缺点 - **优点**: - 简单易用 - 可以同时处理多个任务 - **缺点**: - GIL(全局解释者锁)的存在限制了多线程在某些场景下的性能提升 ### multiprocessing模块 multiprocessing模块用于在不同的进程中运行函数,适用于需要绕过GIL限制的场景。 ```python import multiprocessing def task(): print(f"Process {multiprocessing.current_process().name} is running") if __name__ == "__main__": for i in range(5): process = multiprocessing.Process(target=task, name=f"Process-{i+1}") process.start() print("主进程结束") ``` ## asyncio:Python的异步编程解决方案 ### 事件循环与协程 asyncio库通过事件循环实现异步编程。开发者可以通过定义协程(coroutine)来处理异步任务。 ```python import asyncio async def task(): await asyncio.sleep(1) print(f"Task {asyncio.current_task().name} completed") async def main(): tasks = [task(), task(), task()] await asyncio.gather(*tasks) if __name__ == "__main__": asyncio.run(main()) ``` ### 协程与多任务处理 协程允许在同一事件循环中运行多个异步任务,通过非阻塞的方式提升系统性能。 ```python async def fetch_data(url): # 模拟网络请求 await asyncio.sleep(2) return f"Data from {url}" async def main(): urls = ["https://example.com/1", "https://example.com/2", "https://example.com/3"] tasks = [fetch_data(url) for url in urls] results = await asyncio.gather(*tasks) print(results) if __name__ == "__main__": asyncio.run(main()) ``` ## 实际案例与解决方案 ### 案例1:Web服务器中的异步处理 在基于asyncio的Web框架(如AIOHTTP)中,开发者可以轻松实现高效的网络服务。 ```python import aiohttp from aiohttp import web async def handle(request): await asyncio.sleep(1) return web.Response(text="Hello, Asyncio!", status=200) async def main(): app = web.Application() app.add_routes([web.get('/', handle)]) runner = web.AppRunner(app) await runner.setup() webbrowser.open("http://localhost:8080") await asyncio.sleep(10000) if __name__ == "__main__": asyncio.run(main()) ``` ### 案例2:网络爬虫的异步处理 在爬虫任务中,使用asyncio可以显著提高数据抓取的速度。 ```python import asyncio async def fetch(url): async with aiohttp.ClientSession() as session: response = await session.get(url) return await response.text() async def main(): urls = ["https://example.com/1", "https://example.com/2", "https://example.com/3"] tasks = [fetch(url) for url in urls] results = await asyncio.gather(*tasks) print(results) if __name__ == "__main__": asyncio.run(main()) ``` ## 异步编程的常见问题与解决方案 ### 1. GIL的影响 由于Python的GIL,多线程在计算密集型任务中并不能显著提升性能。对于此类场景,可以考虑使用多进程。 ### 2. 协程的适用场景 协程适用于IO密集型任务(如网络请求、文件读写等)。对于计算密集型任务,建议结合多进程和asyncio使用。 ## 总结 - **同步与异步**:理解两者的区别和应用场景是掌握异步编程的基础。 - **多线程与multiprocessing模块**:了解各自的优缺点,并在合适的场景下选择合适的方法。 - **asyncio库的应用**:通过事件循环和协程实现高效的异步任务处理,特别是在IO密集型场景中表现优异。 通过合理使用Python的异步编程功能,开发者可以显著提升应用的性能和响应速度。 ------ ***操作记录*** 作者:LY小助手 操作时间:2025-03-17 02:20:22 【时区:Etc/UTC】 事件描述备注:使用码本API,保存/发布 地球 [](如果不需要此记录可以手动删除,每次保存都会自动的追加记录)